Deep Dive into TypeScript Decorators
Introduction
TypeScript decorators are a powerful feature that allows you to add metadata to classes, methods, properties, and parameters. They provide a way to modify or enhance the behavior of your code without changing its structure.
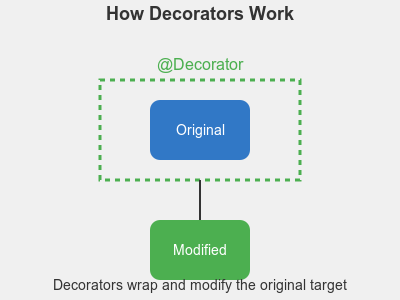
Decorators are a stage 3 proposal for JavaScript and are available as an experimental feature in TypeScript. They are functions that can be attached to classes, methods, properties, or parameters to modify their behavior or add metadata.
To use decorators in TypeScript, you need to enable the experimentalDecorators compiler option in your tsconfig.json:
Class Decorators
Class decorators are applied to the constructor of the class and can be used to observe, modify, or replace a class definition.
Example: Singleton Pattern
This is a class decorator that implements the Singleton pattern. It returns a new class that extends the original constructor. The new class ensures only one instance is created by checking if an instance already exists before creating a new one.
This is the class we're applying the Singleton pattern to. It has a private constructor (to prevent direct instantiation) and a connect method
This demonstrates the Singleton behavior. Despite trying to create two instances with different names, we end up with the same instance. Both db1 and db2 refer to the same object (the first one created), which is why they both connect to "MySQL".
Method Decorators
Method decorators are applied to the Property Descriptor for the method, allowing you to observe, modify, or replace a method definition.
Example: Memoization
Memoization is an optimization technique where the result of a function is cached, so if the same inputs are passed again, the cached result is returned instead of recalculating it, improving performance by avoiding redundant computations.
Decorator Factory:
This is a decorator factory, which is a function that returns the actual decorator. Think of it as a way to customize our decorator before applying it. The decorator function it returns is the real workhorse, taking three important parameters:
Parameter | Description |
---|---|
target | The prototype of the class (for instance methods) or the constructor (for static methods). |
propertyKey | The name of the method being decorated. |
descriptor | An object that describes the method being decorated. |
Memoization Logic:
This is the heart of our memoization technique. We begin by storing the original method and setting up a cache using a Map. Then, we replace the original method with a new function that does some clever work. It turns the arguments into a string key, checks if we've seen these arguments before in our cache, and if so, returns the stored result. If it's a new set of arguments, it calls the original method, saves the result for future use, and returns it. This way, we avoid unnecessary recalculations. Lastly, we return the modified descriptor, completing our memoization process.
Usage of the Memoize Decorator:
Property Decorators
Property decorators are powerful tools that let you enhance or alter how a class property behaves. They act like watchful guardians, allowing you to observe when a property is accessed or changed, and even modify its behavior. This can be incredibly useful for adding validation, logging, or transforming data automatically whenever the property is used.
Example: Validation
This MinLength decorator ensures that the username property always has a minimum length, throwing an error if the requirement is not met.
Usage of the MinLength Decorator:
Conclusion
TypeScript decorators are a powerful feature that can significantly enhance your code's functionality and readability. From implementing design patterns like Singleton to adding validation and memoization, decorators offer a clean and reusable way to modify or extend your classes and methods. While they're still an experimental feature, their potential to simplify complex logic and promote better code organization is immense. As you've seen in these examples, decorators can be incredibly versatile, allowing you to write more expressive and maintainable TypeScript code.