Advanced AWS Lambda Invocations with Node.js
Introduction
AWS Lambda is at the heart of building serverless applications on AWS. It lets us run our code without worrying about servers, but the way we trigger our Lambda functions, and how we connect them with other AWS services, can have a big impact on our system's performance and scalability.
In this post, we'll explore the different Lambda invocation types, dive into event source mappings, and walk through advanced usage patterns with Node.js. We'll also see practical examples, learn best practices, and pick up tips for deploying in the real world.
Lambda Invocation Types
There are two main ways to invoke a Lambda function:
- Synchronous (RequestResponse): The caller waits for the function to finish and gets the result immediately. Used by API Gateway, SDK calls, etc.
- Asynchronous (Event): The caller hands off the event and moves on. Lambda processes it later. Used by S3, SNS, EventBridge, etc.
Let's see both in action with Node.js:
In the following example, we demonstrate how to invoke a Lambda function synchronously from another Lambda function. With synchronous invocation, the caller waits for the function to complete execution and receive the response before continuing.
In the following example, we invoke a Lambda function asynchronously using the 'Event' invocation type. With asynchronous invocation, we don't wait for or expect a response cuz Lambda processes the event in the background and returns immediately.
Event Source Mapping
Event Source Mapping is how Lambda connects to event sources like SQS, DynamoDB Streams, and Kinesis. AWS automatically polls these sources and invokes our function with batches of events. This is the secret sauce for building event-driven architectures
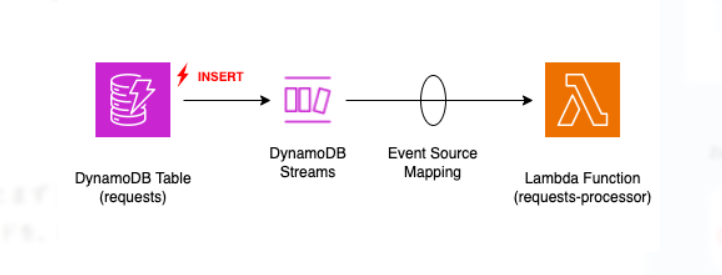
We can create event source mappings via the AWS Console, CLI, or programmatically:
Handling Batched Events in Node.js
When Lambda is triggered by SQS, DynamoDB Streams, or Kinesis, it receives a batch of records:
Advanced: Partial Batch Response (SQS)
With SQS, we can avoid reprocessing successful messages in a failed batch by returning a partial batch response:
Advanced Invocation Patterns
AWS Lambda offers several sophisticated patterns for building complex serverless applications. You can create modular workflows by chaining Lambda functions together, where one function directly invokes another to create a sequence of operations. For parallel processing needs, EventBridge enables fan-out patterns where a single event can trigger multiple Lambda functions simultaneously, allowing for efficient distributed processing.
When you need more control over your function workflows, AWS Step Functions provides powerful orchestration capabilities. It allows you to coordinate multiple Lambda functions with built-in error handling and automatic retries, making it ideal for complex business processes. For improved reliability, you can implement error handling strategies using Dead Letter Queues (DLQs) and custom retry logic, ensuring your applications remain resilient even when issues occur.
Additionally, Lambda supports custom invocation contexts, allowing you to pass metadata for various purposes such as distributed tracing, feature flags, and other application-specific needs. This flexibility enables you to build sophisticated monitoring and control mechanisms into your serverless applications.
Chaining Lambdas Example
Here's an example of chaining Lambda functions, where one function invokes another. This pattern is useful when you need to break down complex operations into smaller, more manageable steps. The first Lambda function can process data and then pass the results to a second function for further processing. This creates a sequential workflow where functions execute one after another.
Fan-out with EventBridge
EventBridge enables powerful fan-out patterns where a single event can trigger multiple Lambda functions simultaneously. This is particularly useful for scenarios where you need parallel processing or want to decouple different parts of your application. For example, when a new user signs up, you might want to simultaneously send a welcome email, create a user profile, and trigger analytics tracking all as separate Lambda functions processing the same event. The following example shows how to publish an event that multiple Lambda functions can subscribe to and process independently.
Step Functions for Orchestration
We can use Step Functions to coordinate multiple Lambdas with error handling and retries.
Error Handling and DLQs
We can configure Dead Letter Queues (DLQs) and custom retry logic for failed events.
Node.js Lambda Invocation Patterns
When working with AWS Lambda functions in Node.js, there are several key invocation patterns we need to understand. First, we can programmatically invoke Lambda functions using the AWS SDK, which gives us fine-grained control over function execution. The SDK allows us to specify invocation types and pass custom payloads to our functions. We also have access to the powerful context object, which provides important metadata and utilities for handling the function's execution environment. This includes things like the request ID, remaining execution time, and logging capabilities. Additionally, we need robust error handling patterns to gracefully handle failures and ensure our functions are resilient. Finally, Lambda supports parallel invocations, enabling us to execute multiple functions concurrently for better performance and scalability in distributed architectures.
Best Practices
To follow best practices with AWS Lambda, make sure to use environment variables for configuration settings. For event-driven Lambdas, implement idempotency to avoid processing the same event more than once. Set up monitoring with CloudWatch Logs and X-Ray to track performance and troubleshoot issues.
Always secure your functions using least privilege IAM roles. For handling errors in asynchronous invocations, use Dead Letter Queues (DLQs). When working with event source mappings, fine-tune batch size and concurrency settings for optimal performance. If you're using SQS as a trigger, enable partial batch responses to prevent message loss or duplication.
Conclusion
Understanding how Lambda invocation types and event source mappings work is key to getting the most out of AWS serverless. With Node.js, we have the tools to build systems that are scalable, responsive, and easy to maintain.